Software Licensing – Updater Implementation for WordPress Plugins
Included with your Software Licensing extension purchase is a sample plugin, which is found in the samples directory inside the Software Licensing zip file. Download the extension from your Account page on our website. It is highly recommended that you open this up and look at it in its entirety.
There are two components to the updater:
- The EDD_SL_Plugin_Updater.php file. This contains the class that makes the magic happen.
- The code snippet that you place somewhere in your plugin that loads the updater provided by the EDD_SL_PLugin_Updater.php class file. This code is in the edd-sample-plugin.php file.
Step 1 – Define the updater constants
In your main plugin file, preferably near the top, or where you have other constants defined, add the following code:
// this is the URL our updater / license checker pings. This should be the URL of the site with EDD installed define( 'EDD_SAMPLE_STORE_URL', 'https://yoursite.com' ); // IMPORTANT: change the name of this constant to something unique to prevent conflicts with other plugins using this system // the download ID. This is the ID of your product in EDD and should match the download ID visible in your Downloads list (see example below) define( 'EDD_SAMPLE_ITEM_ID', 123 ); // IMPORTANT: change the name of this constant to something unique to prevent conflicts with other plugins using this system
EDD_SAMPLE_STORE_URL must be set to the URL of your site that has Easy Digital Downloads installed.
EDD_SAMPLE_ITEM_ID must be set to the ID of your product in EDD. Every product has a unique number ID in your admin area. You can find this in your WordPress dashboard when editing products:
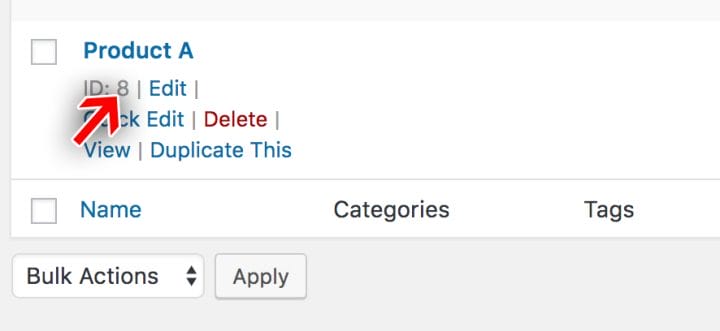
Step 2 – Include the updater class
Recommended: As more and more plugin developers use the Software Licensing extension to deliver updates to their products, there is a risk of a “conflict” if another plugin includes a different version of the EDD_SL_Plugin_Updater class than you are packaging with your plugin. You can rename this class to something unique to your plugin so that it avoids conflicts. For instance, if my plugin name is “My Custom Ads”, I could rename `EDD_SL_Plugin_Updater` to `MCA_Plugin_Updater` and change any references to it.
The update system itself is contained within the file called “EDD_SL_Plugin_Updater.php”. This file can be found in the Software Licensing plugin folder inside samples/edd-sample-plugin/. You need to copy this file into your own plugin’s folder. It can be in the same main folder as your plugin file, or in a sub-directory like /includes/. Either way, you need to refer to it properly in the code, like so:
if ( ! class_exists( 'EDD_SL_Plugin_Updater' ) ) { // load our custom updater if it doesn't already exist include dirname( __FILE__ ) . '/EDD_SL_Plugin_Updater.php'; }
You may need to adjust the file path, depending on where you have decided to place the file within your plugin’s folder structure.
Step 3 – Instantiate the updater class
We now instantiate our updater class like this:
// retrieve our license key from the DB $license_key = trim( get_option( 'edd_sample_license_key' ) ); // setup the updater $edd_updater = new EDD_SL_Plugin_Updater( EDD_SAMPLE_STORE_URL, __FILE__, array( 'version' => '1.0', // current version number 'license' => $license_key, // license key (used get_option above to retrieve from DB) 'item_id' => EDD_SAMPLE_ITEM_ID, // id of this plugin 'author' => 'Author Name', // author of this plugin 'beta' => false // set to true if you wish customers to receive update notifications of beta releases ) );
The license key in this example is stored in an option called “edd_sample_license_key”. You will need to adjust this to retrieve the license key from the option you have set up for your plugin. If you need help setting up an option to store the license key, take a look at the sample plugin, as it includes a fully functional settings page.
There are several parameters we pass to the EDD_SL_Plugin_Updater class:
- $api_url – this is the URL of your site that is running Easy Digital Downloads (and the Software Licensing add-on). Pass the EDD_SAMPLE_STORE_URL constant we defined earlier here.
- $plugin_file – this is the main plugin file. We suggest you use the __FILE__ magic constant provided by PHP. Note, that to do this, you must have this snippet placed in your main plugin file.
- $api_args – this is an array of options to pass to the updater:
- version – this is the current version of the plugin that is installed. It is not the latest available version.
- license – this is the license key retrieved from the database.
- item_id – this is the ID of our product in Easy Digital Downloads. Just pass the EDD_SAMPLE_ITEM_ID constant we defined earlier here.
- author – this is the name of the person or company that wrote the plugin (you!)
- beta – this allows you to indicate if customers should receive update notifications for beta version. See Releasing beta versions for more information.
Note: beginning with Software Licensing version 3.6.12, the plugin updater class is at version 1.8, and includes documented support for auto updates, which WordPress added in version 5.5. To support auto updating, you will need to make sure your updater call is loaded on the `init` hook. Previously, the sample code recommended using `admin_init`.
The sample plugin/theme within the Software Licensing extension should be considered the authoritative source for sample code.
Step 4 – Create a settings page
For a plugin to receive updates, the license key needs to be activated. To activate a license key, the customer will need to enter the key in a field within your plugin settings; then that key needs to be sent to the Software Licensing API on your store’s site.
The sample plugin included with Software Licensing uses a settings page with a single input field. This can work quite well but is meant primarily for demonstrative purposes. It is recommended that you integrate the license key field on your existing settings pages.
This code sets up a submenu page called “Plugin License” under the Plugins menu.
There are two lines at the top of the edd_sample_license_page function:
$license = get_option( 'edd_sample_license_key' ); $status = get_option( 'edd_sample_license_status' );
The first is the license itself and the second is the status of the license. Once we have activated our license, we will change the status (on our local site) to “active”. This is so that we can show an “Activate License” button if the license has not yet been activated, and hide the button if it has. See the screenshot below:
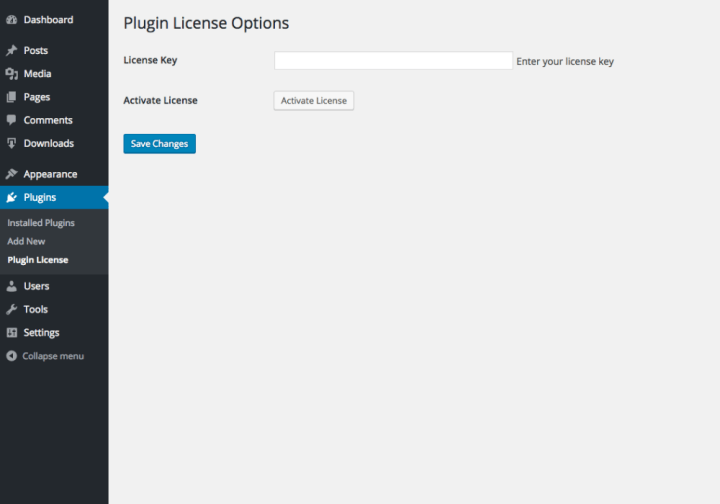
The idea here is that we first enter a license key and click “Save Changes”, which causes the license key to be stored in our plugin/theme options. Once the option is stored, we click the “Activate License” button to trigger the API call.
The activate button is just a simple input field with a type of “submit” and a name attribute that is different than our save button. The names must be different so that we can know when the activate license button was clicked.
Step 5 – Activate the license key
To activate a license key, we “listen” for the Activate License button to be clicked then grab the value entered in the license key field and send an activation request to the API.
If everything runs correctly after clicking the “Activate License” button, the activate button will be replaced with the word “active”, and the license status will reflect the newly activated state in your EDD store’s dashboard. If there is an error when activating the license key, the page will be reloaded and an error and message parameter will be added to the URL. We can then use the admin_notices hook (or any other applicable method) to display the error to the customer.
For example, if a customer enters an invalid license key, an error message will be displayed:
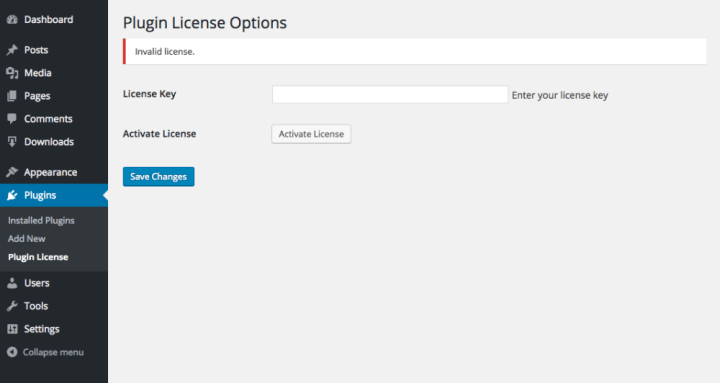
That’s it!
Important notes
- The code shown on this page is purely for demonstrative purposes and is not meant to be used as is. Do not copy and paste it into your plugin and expect it to work as is.
- All function names added to your plugin need to get a unique prefix. We have used edd_sample_ throughout this example. Do not keep edd_sample_ in your own plugin, replace it with your own unique prefix.
- The names of the constants must be changed. Do not keep them as EDD_SAMPLE_ITEM_ID and EDD_SAMPLE_STORE_URL. Use your own unique constants.
- If you are extending the updater class from a file other than the main plugin file, add a define( ‘YOUR_PREFIX_PLUGIN_FILE’, __FILE__ ); to the main plugin file, and then instead of calling __FILE__ as the second parameter of the new EDD_SL_Plugin_Updater class, call YOUR_PREFIX_PLUGIN_FILE there.
- If you have issues with SSL verification when requesting updates, you can use the `edd_sl_api_request_verify_ssl` filter to disable the SSL Verification flag.
- WordPress 5.5 introduced auto-updates for themes and plugins. If you would like to prevent your users from enabling auto-updates for your theme or plugin, you can add a snippet to your distributed code to disable auto-updates:
add_filter( 'auto_update_plugin', 'edd_sample_disable_plugin_autoupdates', 10, 2 );
function edd_sample_disable_plugin_autoupdates( $update, $plugin ) {
if ( 'my-plugin/my-plugin.php' === $plugin->plugin ) {
return false;
}
return $update;
}
add_filter( 'auto_update_theme', 'edd_sample_disable_theme_autoupdates', 10, 2 );
function edd_sample_disable_theme_autoupdates( $update, $theme ) {
if ( 'my-theme' === $theme->theme ) {
return false;
}
return $update;
}